Back in June a developer asked a C++Builder question on StackOverflow. The question was “How can I list all the available VCL styles in a combo box and in the ComboBoxChange event, apply that style in C++ Builder?” I answered the question and gave the example code.
One of my favorite RTL features for the VCL and FMX frameworks is the ability to customize the look and feel of your applications using Styles. The first step is to create a C++Builder VCL project and select a few of the VCL Styles in the Project | Options | Application | Appearance settings. The C++Builder 10.4 Sydney DocWiki explains that “the VCL Styles architecture has been significantly extended to support High-DPI graphics and 4K monitors. In 10.4 Sydney all graphical elements are automatically scaled for the proper resolution of the monitor the element is displayed on. This means that the scaling depends on the DPI resolution of the target computer or the current monitor, in case of multi-monitor systems.”
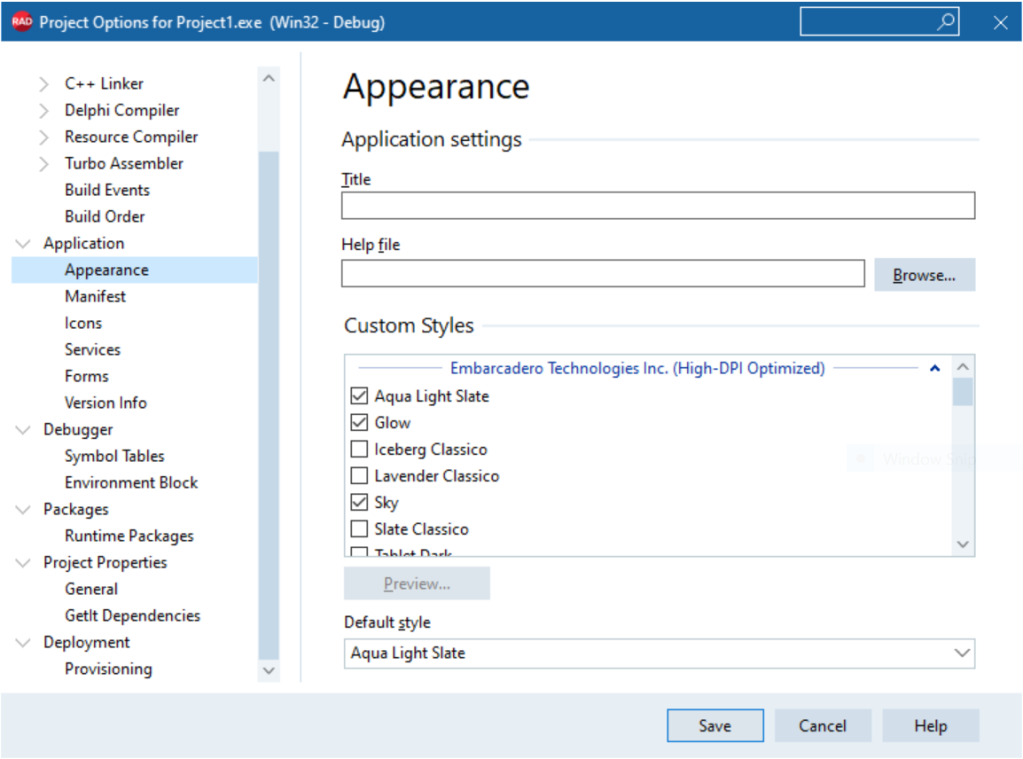
The C++ Application User Interface
For the application user interface I have a TButton (has code to populate the ComboBox with selected application styles) and a TComboBox (to display and allow selection of a style).
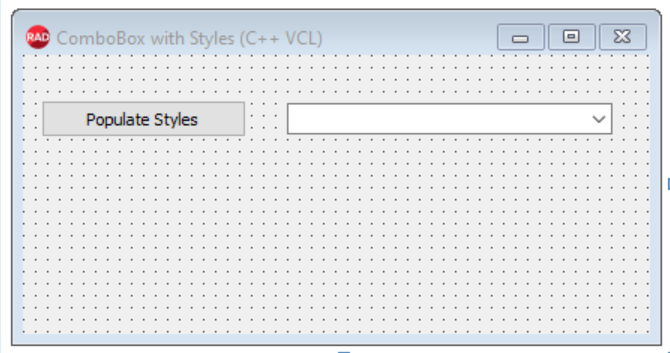
The C++ code
//---------------------------------------------------------------------------
#include <vcl.h>
#pragma hdrstop
#include "Unit1.h"
#include <Vcl.Themes.hpp>
//---------------------------------------------------------------------------
#pragma package(smart_init)
#pragma resource "*.dfm"
TForm1 *Form1;
//---------------------------------------------------------------------------
__fastcall TForm1::TForm1(TComponent* Owner)
: TForm(Owner)
{
}
//---------------------------------------------------------------------------
void __fastcall TForm1::Button1Click(TObject *Sender)
{
// populate the ComboBox with VCL styles that are selected
// in Project | Options | Application | Appearance
ComboBox1->Items->BeginUpdate();
try
{
ComboBox1->Items->Clear();
DynamicArray<String> styleNames = Vcl::Themes::TStyleManager::StyleNames;
for(int i = 0; i < styleNames.Length; ++i)
{
String styleName = styleNames[i];
ComboBox1->Items->Add(styleName);
}
}
__finally
{
ComboBox1->Items->EndUpdate();
}
}
//---------------------------------------------------------------------------
void __fastcall TForm1::ComboBox1Change(TObject *Sender)
{
// set the style for the selected combobox item
Vcl::Themes::TStyleManager::TrySetStyle(ComboBox1->Items->Strings[ComboBox1->ItemIndex],false);
}
//---------------------------------------------------------------------------
The Application in Action


References
Project Options Application Appearance
VCL Styles Support for High-DPI Graphics
C++Builder Product Information
C++Builder Product Page – Native Apps that Perform. Build Windows C++ Apps 10x Faster with Less Code
C++Builder Product Editions – C++Builder is available in four editions – Professional, Enterprise, Architect and Community (free). C++Builder is also available as part of the RAD Studio development suite.